Search View
Search View is where users can search for stickers with search tags like happy, sad, what!, and more and find stickers they can send on chat. You can think of it like the GIF search engine, but optimized for stickers. The sticker search engine will get you the best results from 150,000+ stickers. By adding a single line of code, you'll be able to add this feature to your app, and customize the UI to make it better.
Components
Users can use the 3 main components within the Search View to browse stickers for instant usage:
• Search Bar: Enter search tags to search for stickers.
• Recommended Tag: Click on a recommended tag for quicker use.
• Results window: Tap on a sticker to send it to chat.
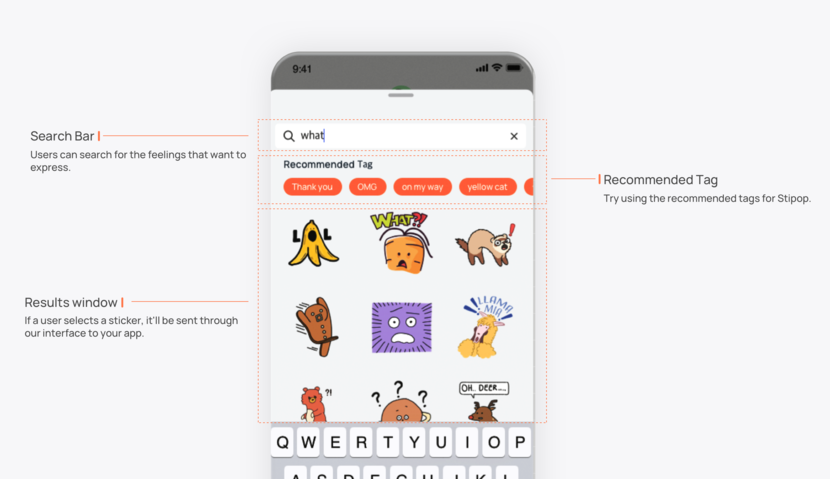
Before You Begin
Make sure you went through all processes in Before You Begin to download and setup the Stipop.plist file, which is required to complete development of the SDK.
1. Make a UIView Object on Storyboard
Go to the Storyboard or Xib you want to place the Search View. Make an object and link it with @IBOutlet like video below.
2. Set SPUISearchViewController
Now, make SPUISearchViewController and connect to the searchView you've just made.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
import UIKit
import Stipop
class ViewController: UIViewController {
@IBOutlet weak var searchView: UIView!
let searchViewController = SPUISearchViewController()
override func viewDidLoad() {
super.viewDidLoad()
let user = SPUser(userID: "some_user_id")
searchViewController.setUser(user)
searchViewController.delegate = self
self.addChild(searchViewController)
self.searchView.addSubview(searchViewController.view)
searchViewController.view.frame = self.searchView.bounds
searchViewController.view.autoresizingMask = [.flexibleWidth, .flexibleHeight]
}
}
extension ViewController: SPUIDelegate {
func onStickerSingleTapped(_ view: SPUIView, sticker: SPSticker) {
// This function will be executed when user chooses a sticker.
}
/* If you want to use double tap feature, change the plist file and implement this function. */
func onStickerDoubleTapped(_ view: SPUIView, sticker: SPSticker) {
// This function will be executed when user chooses a sticker.
}
}
3. How to Show Sticker Image
Refer Quick Implementation - 3. How to Show Sticker Image
1
2
3
4
5
6
7
8
func onStickerSingleTapped(_ view: SPUIView, sticker: SPSticker) {
stickerView.setSticker(sticker)
}
/* If you use double tap feature, this will work too. */
func onStickerDoubleTapped(_ view: SPUIView, sticker: SPSticker) {
stickerView.setSticker(sticker) // This function will be executed when user chooses a sticker.
}
4. Done
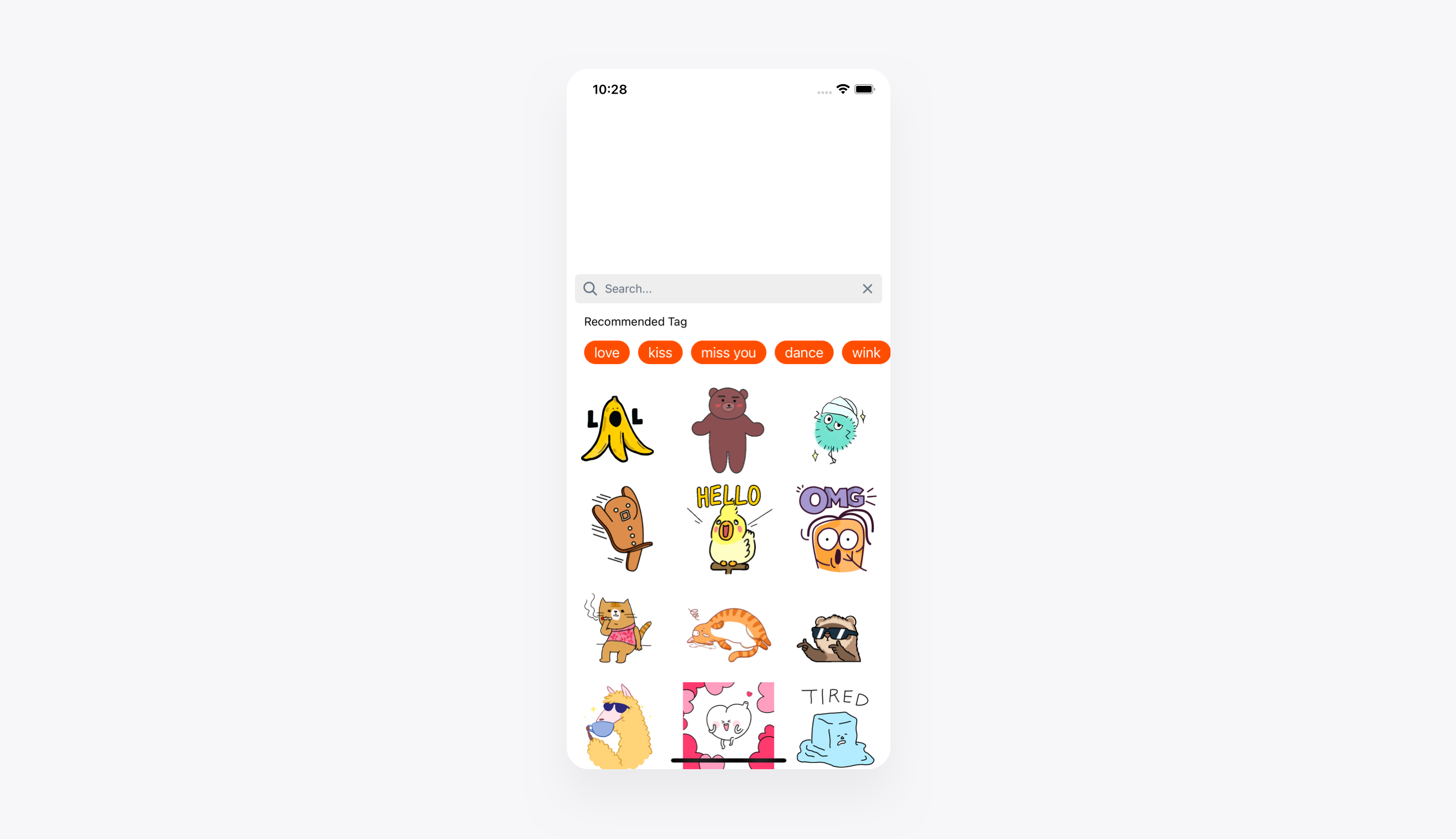
Wonderful! Now, you can implement Search View in your app.
Next step: Try out Sticker Picker View too
Now that you've got the Search View done, how about checking out Sticker Picker View?