Sticker Picker View UIKit
Sticker Picker View provides in-depth sticker experience. Instead of instantaneous usage from the Search View, users can download and own stickers for a more intimate sticker sending experience. It works similar to how stickers work on WhatsApp or Facebook Messenger. Users open up sticker picker and tap on stickers to send directly on chat, and click on 'sticker store' to browse more stickers and download to have them on sticker picker.
The Sticker Lite Store comes with over 150,000+ stickers that are curated automatically and updates with new stickers every week. By adding a single line of code, you'll be able to add this feature to your app, and customize the UI to make it better.
Components
Users can use the 4 main Store View components for intimate sticker usage:
• Sticker Navigation: Navigate through my sticker packs and tap on one.
• Sticker Picker: Tap on a sticker to send it to chat.
• Sticker Search: Search for sticker packs or sticker creators.
• Sticker list: Browse the most popular stickers to download and add to my sticker picker.
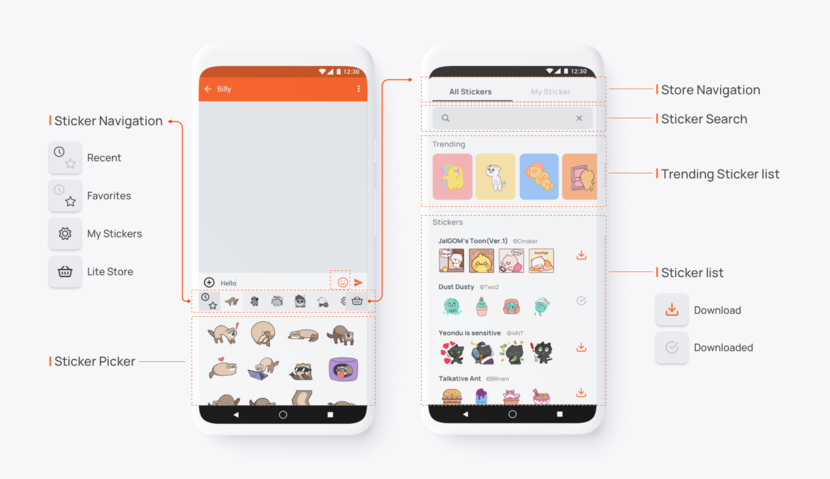
Before You Begin
Make sure you went through all processes in Before You Begin to download and setup the Stipop.plist file, which is required to complete development of the SDK.
1. Make a UIView Object on Storyboard
Go to the Storyboard or Xib you want to place the Sticker Picker View. Make an object and link it with @IBOutlet like video below.
*If your Stipop library version is over 0.7.0, type 'SPUIPickerView' to 'SPUIPickerCustomView'.
2. Set SPUIPickerView
Now, link SPUIPickerView with delegate.
(*If your Stipop library version is over 0.7.0, type 'SPUIPickerView' to 'SPUIPickerCustomView')
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
import UIKit
import Stipop
class ViewController: UIViewController {
@IBOutlet weak var pickerView: SPUIPickerView!
override func viewDidLoad() {
super.viewDidLoad()
let user = SPUser(userID: "some_user_id")
pickerView.setUser(user)
pickerView.delegate = self
}
}
extension ViewController: SPUIDelegate {
func onStickerSingleTapped(_ view: SPUIView, sticker: SPSticker) {
// This function will be executed when user chooses a sticker.
}
/* If you want to use double tap feature, change the plist file and implement this function. */
func onStickerDoubleTapped(_ view: SPUIView, sticker: SPSticker) {
// This function will be executed when user chooses a sticker.
}
}
3. How to Show Sticker Image
Refer Quick Implementation - 3. How to Show Sticker Image
1
2
3
4
5
6
7
8
func onStickerSingleTapped(_ view: SPUIView, sticker: SPSticker) {
stickerView.setSticker(sticker)
}
/* If you use double tap feature, this will work too. */
func onStickerDoubleTapped(_ view: SPUIView, sticker: SPSticker) {
// This function will be executed when user chooses a sticker.
}
3. Done
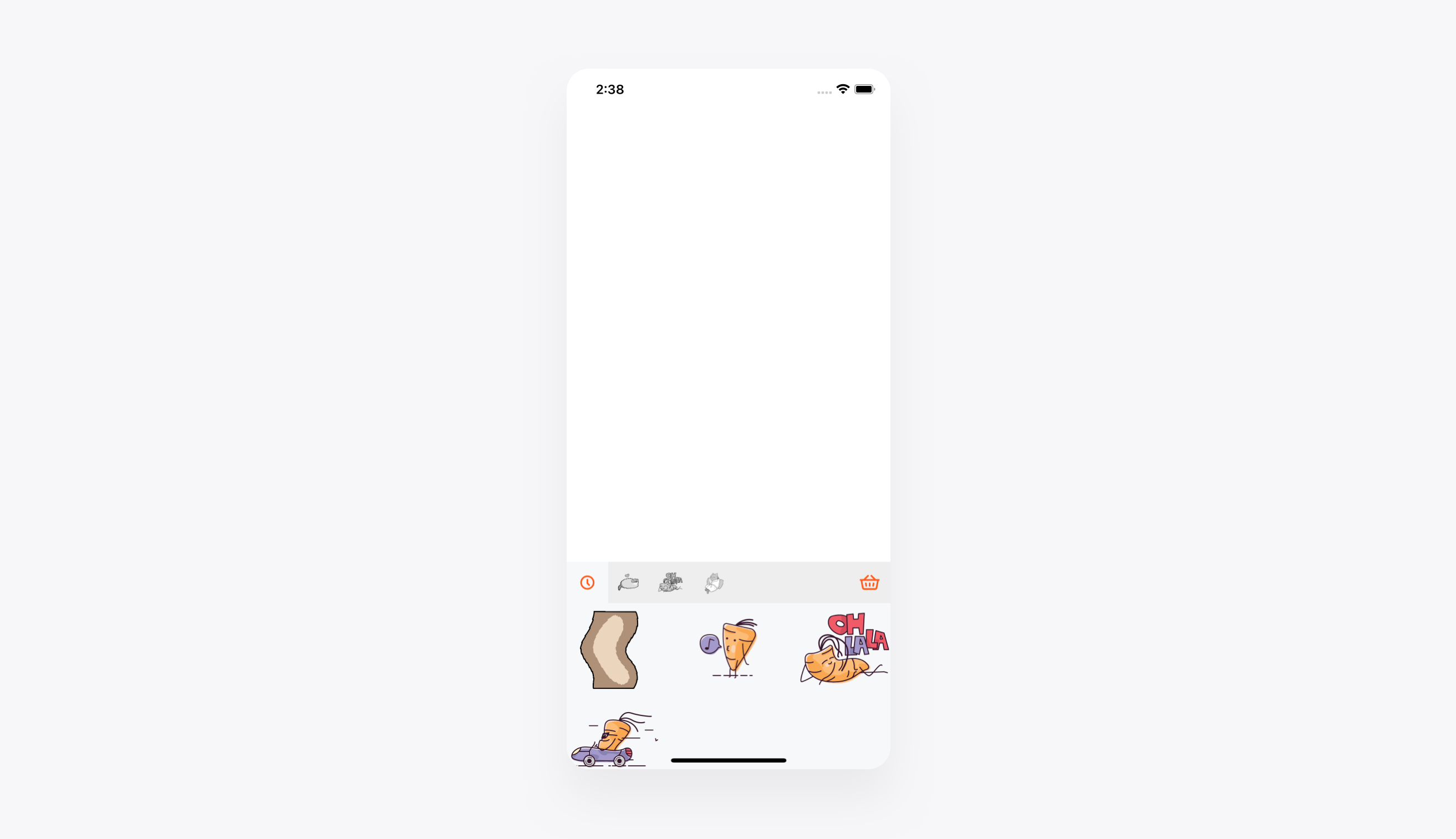
Wonderful! Now, you can implement Sticker Picker View in your app.
Next step: Customize UI
Now that you've got the Sticker Picker View done, let's find out how to customize UI to fit with your app.